Ver versión para PHP
Lo primero que tenemos que hacer es agregar una clase llamada Charts a nuestro proyecto:
using System.Collections.Generic;
public class Charts
{
public List<ChartColumn> cols = new List<ChartColumn>();
public List<ChartRow> rows = new List<ChartRow>();
public struct ChartColumn
{
public string id;
public string label;
public string pattern;
public string type;
public string role;
}
public struct ChartRow
{
public List c;
}
public struct ChartRowValue
{
public object v;
public object f;
}
public void AddColumn(ChartColumn col) { cols.Add(col); }
public void AddRow(ChartRow row) { rows.Add(row); }
}
Esta clase nos servirá para poder generar el Json estandar que requieren las gráficas de Google.
Luego, agregamos una página, en mi caso "pastel.aspx", con el siguiente código:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="pastel.aspx.cs" Inherits="pastel" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>TyroDeveloper</title>
<script src="https://code.jquery.com/jquery-3.2.1.min.js"
integrity="sha256-hwg4gsxgFZhOsEEamdOYGBf13FyQuiTwlAQgxVSNgt4="
crossorigin="anonymous"></script>
<script src="https://www.gstatic.com/charts/loader.js"></script>
<script type="text/javascript">
google.charts.load('current', { 'packages': ['corechart'] });
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
//Pastel
$.ajax({
type: 'POST',
url: "pastel.aspx/GraficaPastel",
dataType: "json",
contentType: 'application/json',
async: false,
success: function (result) {
var data = new google.visualization.DataTable(result.d);
var options = {
title: '',
hAxis: {
title: 'Tipo'
},
vAxis: {
title: 'Total'
},
pointSize: 5,
backgroundColor: { fill: 'transparent' },
'height': 300
};
target = document.getElementById('pie-chart');
var chart = new google.visualization.PieChart(target);
chart.draw(data, options);
}
});
}
</script>
<style>
body {
font-family: Verdana;
}
</style>
</head>
<body>
<form id="frmChart" runat="server">
<div>
<div id="pie-chart"></div>
</div>
</form>
</body>
</html>
Por último, el código C#:
using System;
using System.Collections.Generic;
using System.Web.Script.Serialization;
using System.Web.Services;
public partial class pastel : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[WebMethod]
public static string GraficaPastel()
{
GoogleCharts chart = new GoogleCharts();
//Agregar columnas
chart.cols.Add(new GoogleCharts.ChartColumn() {
id = "",
label = "Tipo",
pattern = "",
type = "string"
});//col1
chart.cols.Add(new GoogleCharts.ChartColumn() {
id = "",
label = "Total",
pattern = "",
type = "number"
});//col2
//Agregar datos
//Agregar 20 Fresas
chart.rows.Add(new GoogleCharts.ChartRow()
{
c = new List<GoogleCharts.ChartRowValue>() {
{ new GoogleCharts.ChartRowValue() { v = "Fresas" } },
{ new GoogleCharts.ChartRowValue() { v = 20 } }
}
});
//Agregar 5 MAnzanas
chart.rows.Add(new GoogleCharts.ChartRow()
{
c = new List<GoogleCharts.ChartRowValue>() {
{ new GoogleCharts.ChartRowValue() { v = "Manzanas" } },
{ new GoogleCharts.ChartRowValue() { v = 5 } }
}
});
return new JavaScriptSerializer().Serialize(chart);
}
}
Así se ve nuestra gráfica:
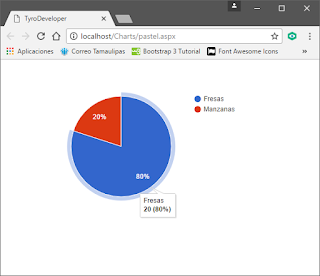
No hay comentarios:
Publicar un comentario