Hoy les voy a mostrar como hacer una gráfica de pastel con Flotcharts y ASP.Net.
Lo primero que necesitamos es una clase C#. Agregamos una clase llamada "PieChart". Nuestra clase estará dentro de un espacio de nombres que llamaremos "FlotCharts", el código es el siguiente:
using System.Collections.Generic;
/// <summary>
/// Descripción breve de FlotCharts
/// </summary>
namespace FlotCharts
{
public class PieChart
{
public List<Serie> series = new List<Serie>();
public struct Serie
{
public string label;
public string color;
public double data;
}
}
}
Ahora, agregamos una página ASP.Net llamada "pastel.aspx", con el siguiente código:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="pastel.aspx.cs" Inherits="flotcharts_pastel" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>TyroDeveloper</title>
<script type="text/javascript" src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/flot/0.8.3/jquery.flot.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/flot/0.8.3/jquery.flot.pie.min.js"></script>
<style>
body {
font-family: Verdana;
}
</style>
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: 'POST',
url: "pastel.aspx/GraficaPastel",
dataType: "json",
contentType: 'application/json',
async: false,
success: function (result) {
var options = {
series: {
pie: {
show: true,
radius: 1,
label: {
show: true,
radius: 1,
formatter: function (label, series) {
return Math.round(series.percent) + '%';
},
background: {
opacity: 0.5,
color: '#C0C0C0'
}
}
}
},
legend: {
show: true
},
grid: {
hoverable: true
}
};
var data = JSON.parse(result.d).series;
$.plot($("#pie-chart"), data, options);
}
});
});
</script>
</head>
<body>
<form id="frmFlotCharts" runat="server">
<div id="pie-chart" style="width: 400px; height: 300px; margin: 0 auto"></div>
</form>
</body>
</html>
Con el siguiente código C#:
using System;
using System.Web.Script.Serialization;
using System.Web.Services;
using FlotCharts;
public partial class flotcharts_pastel : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[WebMethod]
public static string GraficaPastel() {
PieChart chart = new PieChart();
//Agregar 20 Fresas
chart.series.Add(new PieChart.Serie() {
label = "Fresas",
data = 20,
color = "#FF0000"
});
//Agregar 5 Manzanas
chart.series.Add(new PieChart.Serie()
{
label = "Manzanas",
data = 5,
color = "#00CC00"
});
//Generar Json
return new JavaScriptSerializer().Serialize(chart);
}
}
Así se ve nuestra gráfica: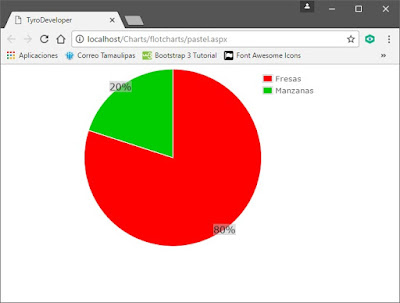
No hay comentarios:
Publicar un comentario