Ahora les voy a mostrar como se hace una gráfica de múltiples líneas con Highcharts y PHP.
Lo primero, nuestra clase "HighCharts.php":
<?php
/**
* Description of HighCharts
*
* @author gabriel.castillo
*/
class HighCharts{
public $categories;
public $data;
}
class HighChartsPie{
public $name;
public $colorByPoint;
public $data;
}
class Serie {
public $name;
public $color;
public $data;
}
class Data{
public $name;
public $y;
public $sliced;
public $selected;
}
?>
Luego de esto, agregamos una página PHP llamada "lineas-multiples.php", con el siguiente código:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>TyroDeveloper</title>
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script src="https://code.highcharts.com/highcharts.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: 'POST',
url: "highcharts-lineas-multiples.php",
dataType: "json",
contentType: 'application/json',
async: false,
success: function (result) {
var options = {
chart: {
plotBackgroundColor: null,
plotBorderWidth: null,
plotShadow: false,
type: 'line',
renderTo: 'line-chart'
},
title: {
text: ''
},
tooltip: {
pointFormat: '{series.name}: <b>{point.y}</b>'
},
plotOptions: {
pie: {
allowPointSelect: true,
cursor: 'pointer',
dataLabels: {
enabled: true
},
showInLegend: true
}
},
legend: {
enabled: true
},
xAxis: {
categories: result.categories,
title: {
text: "Fecha"
}
},
yAxis: {
min: 0,
title: {
text: "Total"
}
},
series: result.data
};
var chart = new Highcharts.Chart(options);
}
});
});
</script>
<style>
body {
font-family: Verdana;
}
</style>
</head>
<body>
<form id="frmChart" runat="server">
<div>
<div id="line-chart" style="min-width: 310px; height: 400px; max-width: 600px; margin: 0 auto"></div>
</div>
</form>
</body>
</html>
Ahora, agregamos un archivo PHP llamado "highcharts-lineas-multiples.php", con el siguiente código:
<?php
include("HighCharts.php");
$chart = new HighCharts();
//inicializar
$chart->categories = array();
$chart->data = array();
//Agregar categorías
array_push($chart->categories, "01/01/2017");
array_push($chart->categories, "02/01/2017");
array_push($chart->categories, "03/01/2017");
array_push($chart->categories, "04/01/2017");
//Agregar serie (Línea)
$serie = new Serie();
$serie->name = "Abarrotes";
$serie->color = "#FF0000";
//Agregar datos de la serie
$serie->data = array();
$serie_data = new Data();
$serie_data->y = 2300.85;
array_push($serie->data, $serie_data);
$serie_data = new Data();
$serie_data->y = 2500;
array_push($serie->data, $serie_data);
$serie_data = new Data();
$serie_data->y = 800;
array_push($serie->data, $serie_data);
$serie_data = new Data();
$serie_data->y = 0;
array_push($serie->data, $serie_data);
//agregar los datos
array_push($chart->data, $serie);
//Agregar serie (Línea)
$serie = new Serie();
$serie->name = "Lácteos";
$serie->color = "#00CC00";
//Agregar datos de la serie
$serie->data = array();
$serie_data = new Data();
$serie_data->y = 750;
array_push($serie->data, $serie_data);
$serie_data = new Data();
$serie_data->y = 1350;
array_push($serie->data, $serie_data);
$serie_data = new Data();
$serie_data->y = 2500;
array_push($serie->data, $serie_data);
$serie_data = new Data();
$serie_data->y = 7000;
array_push($serie->data, $serie_data);
//agregar los datos
array_push($chart->data, $serie);
echo json_encode($chart);
?>
Así se ve nuestra gráfica: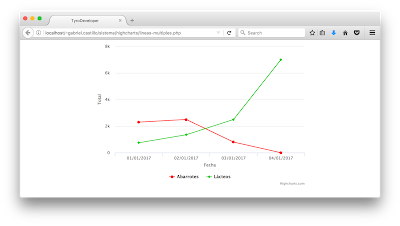
No hay comentarios:
Publicar un comentario